This blog post dives into building a scalable and robust AI news agency by integrating OpenAI’s Swarm library with RabbitMQ. We’ll use the newsq.py
code as a foundation to illustrate how this integration enhances security, scalability, and modularity.
Understanding the AI News Agency
Our news agency utilizes OpenAI’s Swarm library to create an autonomous system for finding and processing AI-related news. Let’s break down the key components:
- Agents: The system consists of several specialized agents, each playing a distinct role:
- NewsDirector: This agent orchestrates the entire process. It determines news topics, assigns tasks to other agents (NewsGatherer, ArticleWriter, Publisher), and ensures content quality.
- NewsGatherer: Responsible for researching and retrieving relevant news articles using the
search_news
function (powered by DuckDuckGo induck.py
). - ArticleWriter: Takes the gathered information and crafts well-structured, engaging articles, adhering to journalistic standards.
- Publisher: Handles the final steps, formatting the article for the target platform (e.g., WordPress) and publishing it.
newsq.py
: This file orchestrates the news agency’s workflow. It defines the agents, their roles, and how they interact.- External Dependencies:
duck.py
: Provides thesearch_news
function, abstracting the DuckDuckGo search functionality.models.py
: Contains a list of available language models that power the agents, offering flexibility in choosing the best model for each task.prompts.py
: Stores the prompts used to instruct and guide the agents, ensuring consistent and effective communication.
Here’s a snippet from newsq.py
demonstrating how the NewsDirector
agent is defined:
news_director = Agent(
name="NewsDirector",
model=model_list[MODEL],
instructions="""You are a News Director responsible for:
1. Deciding what topics to cover
2. Coordinating the news gathering and writing process
3. Ensuring high-quality content
4. Managing the publication workflow
Provide clear instructions about what news to gather and what angle to take.""",
functions=[search_news_agent, write_article, publish_article],
)
Why RabbitMQ Enhances the Agency
While the Swarm library lays the groundwork for agent-based workflows, incorporating RabbitMQ as a message broker brings several significant benefits:
- Scalability: RabbitMQ excels at handling high message throughput, making it ideal for a news agency that might need to process vast amounts of data and agent requests. This asynchronous communication model allows agents to operate independently and scale horizontally as needed.
- Decoupling: RabbitMQ decouples the agents, enhancing system flexibility and maintainability. Agents don’t need to know about each other directly; they only interact through RabbitMQ queues. This separation simplifies adding new agents or modifying existing ones without impacting the entire system.
- Security: RabbitMQ provides robust authentication and authorization mechanisms, ensuring that only authorized agents can publish or consume messages. This is crucial in protecting sensitive data and maintaining the integrity of the news agency’s workflow.
- Fault Tolerance: RabbitMQ’s queuing mechanism provides inherent resilience. If an agent (e.g., the
ArticleWriter
) is temporarily unavailable, messages will queue up, preventing data loss and ensuring continued operation when the agent comes back online.
Implementation Overview
- Message Queues: We can define dedicated queues for communication between agents:
news_requests
: TheNewsDirector
publishes news topic requests to this queue.research_results
: TheNewsGatherer
posts research findings to this queue.writing_tasks
: Articles ready for writing are placed here.publication_queue
: Finalized articles await publication in this queue.
- Agent Integration: Each agent is modified to interact with RabbitMQ:
- The
NewsDirector
publishes messages to thenews_requests
queue. - The
NewsGatherer
consumes fromnews_requests
, performs research, and publishes toresearch_results
. - This pattern continues for other agents, consuming from one queue, processing the message, and publishing results to the next queue.
- The
Code Snippet: RabbitMQ Integration
Here’s an example of how the NewsGatherer
agent could be modified to use RabbitMQ:
import pika
class NewsGatherer:
def __init__(self, rabbitmq_config):
self.connection = pika.BlockingConnection(pika.ConnectionParameters(**rabbitmq_config))
self.channel = self.connection.channel()
# Declare queues
self.channel.queue_declare(queue='news_requests')
self.channel.queue_declare(queue='research_results')
def start_consuming(self):
self.channel.basic_consume(
queue='news_requests', on_message_callback=self.handle_message
)
print('NewsGatherer: Waiting for news requests...')
self.channel.start_consuming()
def handle_message(self, ch, method, properties, body):
news_request = json.loads(body)
# Process news request (e.g., call search_news)
# ...
research_results = {
'status': 'success',
'data': # your research results
}
self.channel.basic_publish(
exchange='', routing_key='research_results', body=json.dumps(research_results)
)
ch.basic_ack(delivery_tag=method.delivery_tag)
Conclusion
Integrating RabbitMQ with OpenAI’s Swarm empowers us to build a highly scalable, robust, and maintainable AI news agency. This combination leverages the power of specialized agents while benefiting from the reliability and efficiency of a dedicated message broker. The provided code examples offer a starting point for your own AI-powered applications, enabling you to build sophisticated systems capable of handling real-world complexities.
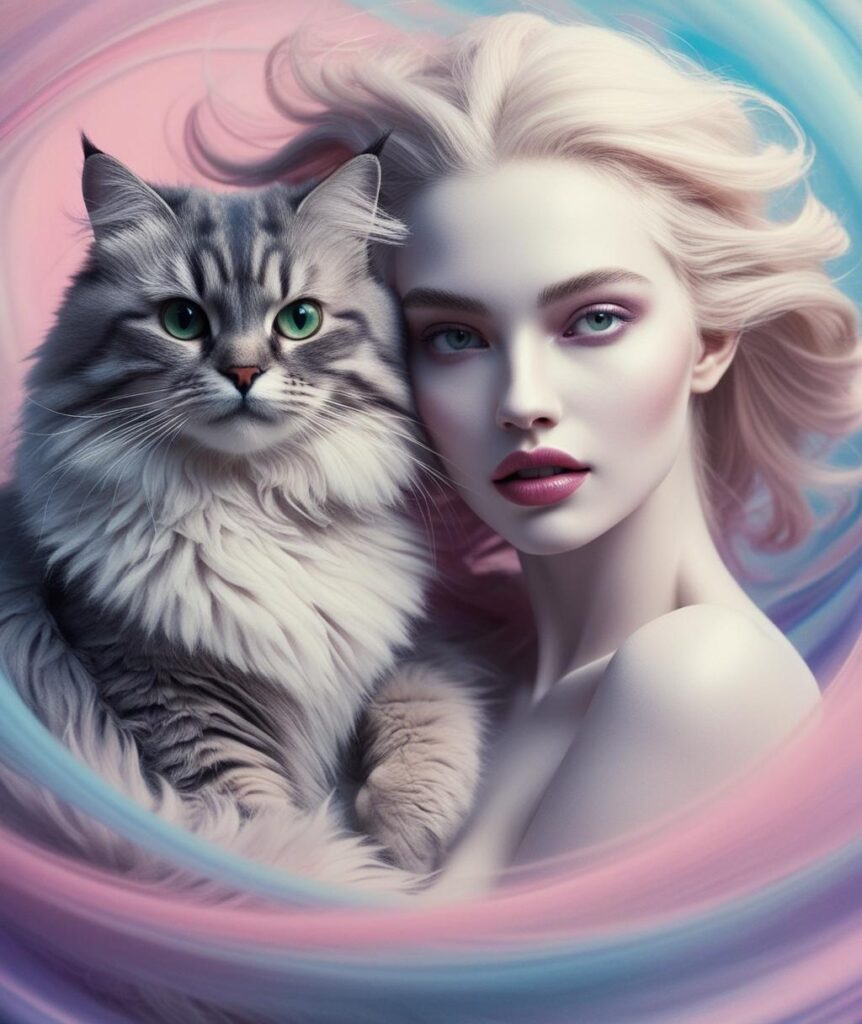